Sahi Pro - Logging APIs
abstract
Logging APIs allow adding additional information to playback logs.
_log
_log($message[, $logType])
Arguments
$message | string | Message to log |
$logType | string optional | Changes the color in which log statement is displayed. Can be one of "INFO" , "SUCCESS" , "FAILURE" ,"CUSTOM" , "CUSTOM1" , "CUSTOM2" , "CUSTOM3" ,"CUSTOM4" , "CUSTOM5" , "CUSTOM6" Defaults to "INFO" |
Details
_log("Current user is " + $user); // Will log as plain text
_log("Current user is " + $user, "CUSTOM1"); // Will log in a different color
_logException
_logException($exception)
Arguments
$exception | Exception | Exception passed to catch block |
Details
_logException is used within a catch block to log the Exception in playback logs.
The logged line will be in plain text and the script status will not be affected by this.
_logException is used within a catch block to log the Exception in playback logs.
The logged line will be in plain text and the script status will not be affected by this.
try{
_click(_link("does not exist"));
}catch($e){
_logException($e); // Logs the exception, but does not fail
}
_logExceptionAsFailure
_logExceptionAsFailure($exception)
Arguments
$exception | Exception | Exception passed to catch block |
Details
_logExceptionAsFailure is used within a catch block to log the Exception in playback logs.
The logged line will be in red and the script will be marked failed because of this.
_logExceptionAsFailure is used within a catch block to log the Exception in playback logs.
The logged line will be in red and the script will be marked failed because of this.
try{
_click(_link("does not exist"));
}catch($e){
_logExceptionAsFailure($e); // Logs the exception, and fails,
// and in the logs, points to the original line as source of failure.
}
Masking logs
_maskLogs
_maskLogs([$message])
Arguments
$message | string optional | Message to print when logging this mask statement |
Details
_maskLogs tells Sahi not to display further steps in the playback logs and in the Controller.
This is used when sensitive information like password may be entered via script, but we do not want it stored/displayed anywhere
Toggled by _unmaskLogs
_maskLogs tells Sahi not to display further steps in the playback logs and in the Controller.
This is used when sensitive information like password may be entered via script, but we do not want it stored/displayed anywhere
Toggled by _unmaskLogs
_unmaskLogs
_unmaskLogs([$message])
Arguments
$message | string optional | Message to print when logging this unmask statement |
Details
_maskLogs tells Sahi not to display further steps in the playback logs and in the Controller.
This is used when sensitive information like password may be entered via script, but we do not want it stored/displayed anywhere
_maskLogs tells Sahi not to display further steps in the playback logs and in the Controller.
This is used when sensitive information like password may be entered via script, but we do not want it stored/displayed anywhere
Example of _maskLogs _unmaskLogs
_setValue(_textbox("user"), "test");
_maskLogs("Password Information start");
_setValue(_password("password"), "secret");
_unmaskLogs("Password Information end");
_click(_submit("Login"));
will show this in logs:
Playback Logs
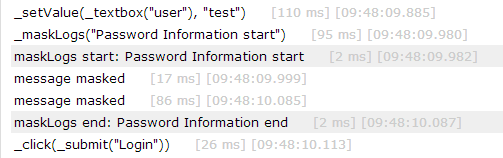
Controller Playback Tab
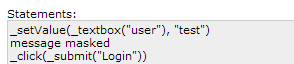
Disable/Enable INFO logging
_disableInfoLogging
_disableInfoLogging()
Arguments
None |
Details
Disables logging of INFO (normal) steps in playback logs.
Only SUCCESS, FAILURE and CUSTOM steps are logged.
Disables logging of INFO (normal) steps in playback logs.
Only SUCCESS, FAILURE and CUSTOM steps are logged.
_enableInfoLogging
_enableInfoLogging()
Arguments
None |
Details
Enables logging of INFO (normal) steps in playback logs
which was previously disabled using
Enables logging of INFO (normal) steps in playback logs
which was previously disabled using
_disableInfoLogging
.Demarcating Test Cases
Sections of a script may need to be marked as separate testcases while viewing in logs.
For example a single script can exercise login, verifyTotal and logout, and may be considered as 3 testcases in a single script.
_testcase
_testcase($testcaseId, $description)
Arguments
$testcaseId | string | Unique identifier for testcase |
$description | string | Description to be shown in logs |
Details
Demarcates a testcase (a logical boundary of steps)
The logs of the above script will look like this:
Also refer to csv files as suites.
Demarcates a testcase (a logical boundary of steps)
_navigateTo("/demo/training/");
var $t = _testcase("TC_1", "Login");
$t.start();
_setValue(_textbox("user"), "test");
_setValue(_password("password"), "secret");
_click(_submit("Login"));
$t.end();
var $t2 = _testcase("TC_2", "Add books").start(); // can be in one line also
_setValue(_textbox("q"), "2");
_setValue(_textbox("q[1]"), "1");
_setValue(_textbox("q[2]"), "1");
_click(_button("Add"));
$t2.end();
var $t3 = _testcase("TC_8", "Verify total");
$t3.start();
_assertExists(_textbox("total"));
_assert(_isVisible(_textbox("total")));
_assertEqual("1150", _textbox("total").value);
$t3.end();
The logs of the above script will look like this:
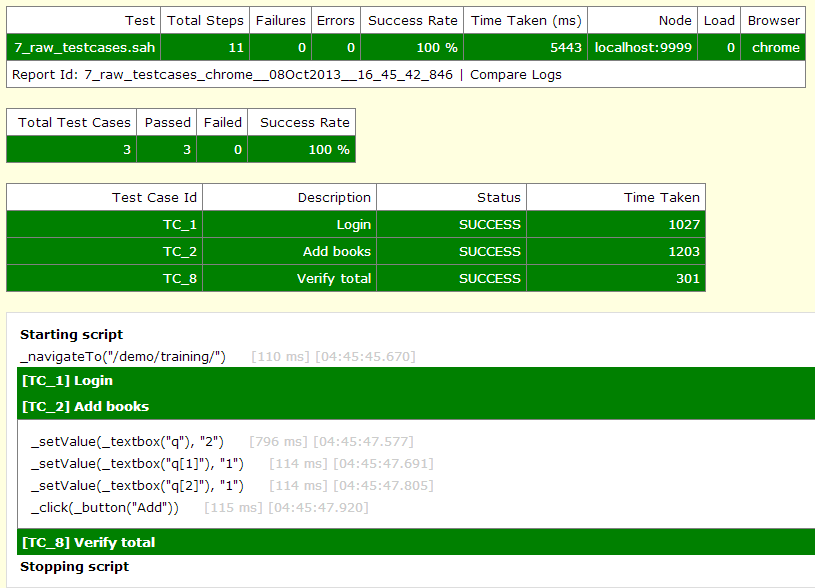
Also refer to csv files as suites.